Sometimes, it may be necessary to display additional information on a web page conditionally facilitated by clicking on a link or icon or button. And, another click to remove (hide) the text on the page.
For example, consider the below simple web page to demonstrate the idea:
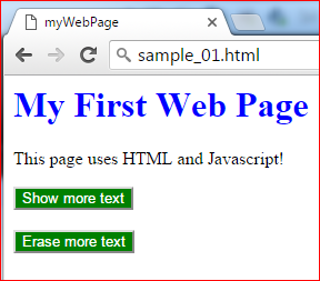
When the button Show more text is clicked, text is added and displayed below the first line after the header. When the Erase more text button is clicked, the text is removed.
Click here to see a live page.
The basis for dynamically adding and removing text on the above demo web page uses Javascript and the HTML DOM innerHTML property,
document.getElementById(“mText”).innerHTML for the element “mText”.
The innerHTML property allows content in element “mText’ to be changed as illustrated in the below code snippet.
<!-- ' '******************************************************** ' My first web page ' ' Author : Elario Belmontes, Jr. ' Description: Web page to demonstrate toggling of text ' using innerHTML property to set HTML content ' of an element ' Languanges : JAVASCRIPT, HTML ' File Name : text_toggle_sample_01.html ' Disclaimer: This software is intended for educational learning ' purposes. ' ' This software is provided "AS IS" and without any ' expressed or implied warranties, including, without ' limitation, the implied warranties of merchantability ' and fitness for a particular purpose. ' ' No guarantee; No warranty; Install / Use at your own risk. ' ' ' Copyright (C) 2016 Larry Belmontes, Jr. ' ' ' '******************************************************** --> <script language="JavaScript"> function moreText() { document.getElementById("mText").innerHTML = document.getElementById("mText").innerHTML + "Free form text on this line...</br>" + "<i>Italicized text here...</i></br>" + "<b>bold text on this line...</b></br></br>"; } function eraseMoreText() { document.getElementById("mText").innerHTML = ""; } </script> <html> <head> <style> h1 {color:blue} input[id^='btn'] {background-color:green; color:white;} </style> <title>myWebPage</title> </head> <body> <h1>My First Web Page</h1> <p>This page uses HTML and Javascript!</p> <!-- Text to be added in the following span area using mText id --> <span id="mText"></span> <input id="btnMoreText" type=button value="Show more text" name="b1" onclick="moreText();"> </br> </br> <input id="btnEraseMoreText" type=button value="Erase more text" name="b2" onclick="eraseMoreText();"> </body> </html>
The above code snippet utilizes Javascript and HTML. The Javascript portion executes on the client-side (your browser) and does not involve sending any data to the hosting server.
Take a look and apply this concept to your next web page!